Recently, I’ve been struggling to find what Public IP resources I have in my Azure Subscription and find out where those IPs are being used and I noticed the Azure portal search doesn’t help in locating the resource level IPs. So, I decided to create a script that can search through all the Public IP resources and find out which IP is assigned to each one of them and search the desired IP.
Let’s dive into the script and see how we can achieve it.
- Environment Setup
- Code
- Conclusion
Environment Setup
You can use VS-Code or PowerShell ISE, or Cloud Shell with PowerShell Module to connect to the subscription and access the resources from terminal.
For PowerShell ISE/VS Code
Download Azure PowerShell module from PS Gallery using following commands.
Install-Module -Name Az -AllowClobber
If you get a scope error, use the following with “-scope” parameter.
Install-Module -Name Az -AllowClobber -Scope CurrentUser
Code
Sign in to Azure
Before running the code, Sign-In to Azure Account using the following command in either VS Code or PowerShell ISE to make sure you have proper permissions to read and query the resources.
Cloud Shell will be automatically authenticated using your Azure Login credentials.
Connect-AzAccount
I’ve distributed the code in functions to make it easier to recall for different scenarios. Let’s look at the following code snippet and see what it does.
Code Breakdown
The code is able to get all the resources and save them locally into a json file for multiple user and avoid repetitive queries on Azure resources.
We initialize $json
object which will store all the resources to query from. $filepath
specify the location of resource info exported by the script to be used as cache. $ipAddress
will be used by the script to get user input with “IP” address spcified in format XX.XX.XX.XX
$context
variable validates that user is logged in and able to query resources from Azure Subscription. This saves us from logging into Azure every time we run the script.
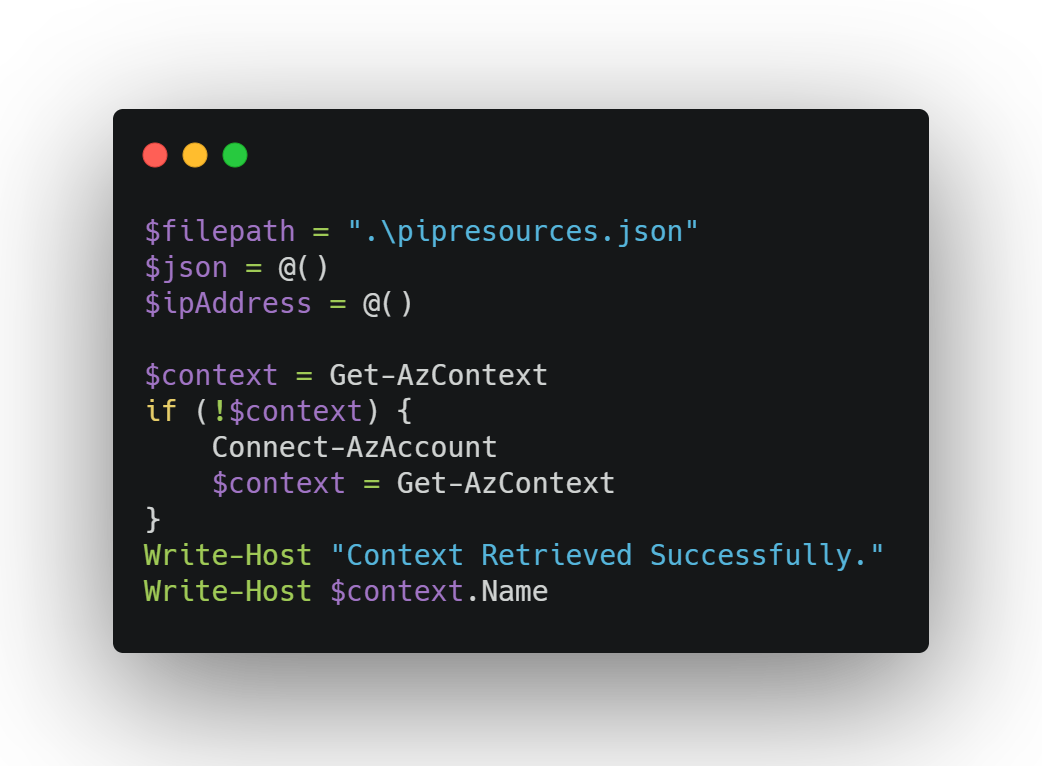
The first function performs the query on Azure resources and get all the Resources with Public IP Address. The if
loop check for context and make sure user is signed in before running any query against the subscription. User will be prompted to login if $context
isn’t received for any reason.
For sake of demo, we will be querying from multiple subscriptions under same tenant and other subscriptions that might be accessible from different tenant by calling Set-AzContext
for each subscription, retrieve the resources and store the data in $allSub
.
All the resources are stored in $pipResource
which are then stored in a file for running multiple queries without pulling data from cloud every time.
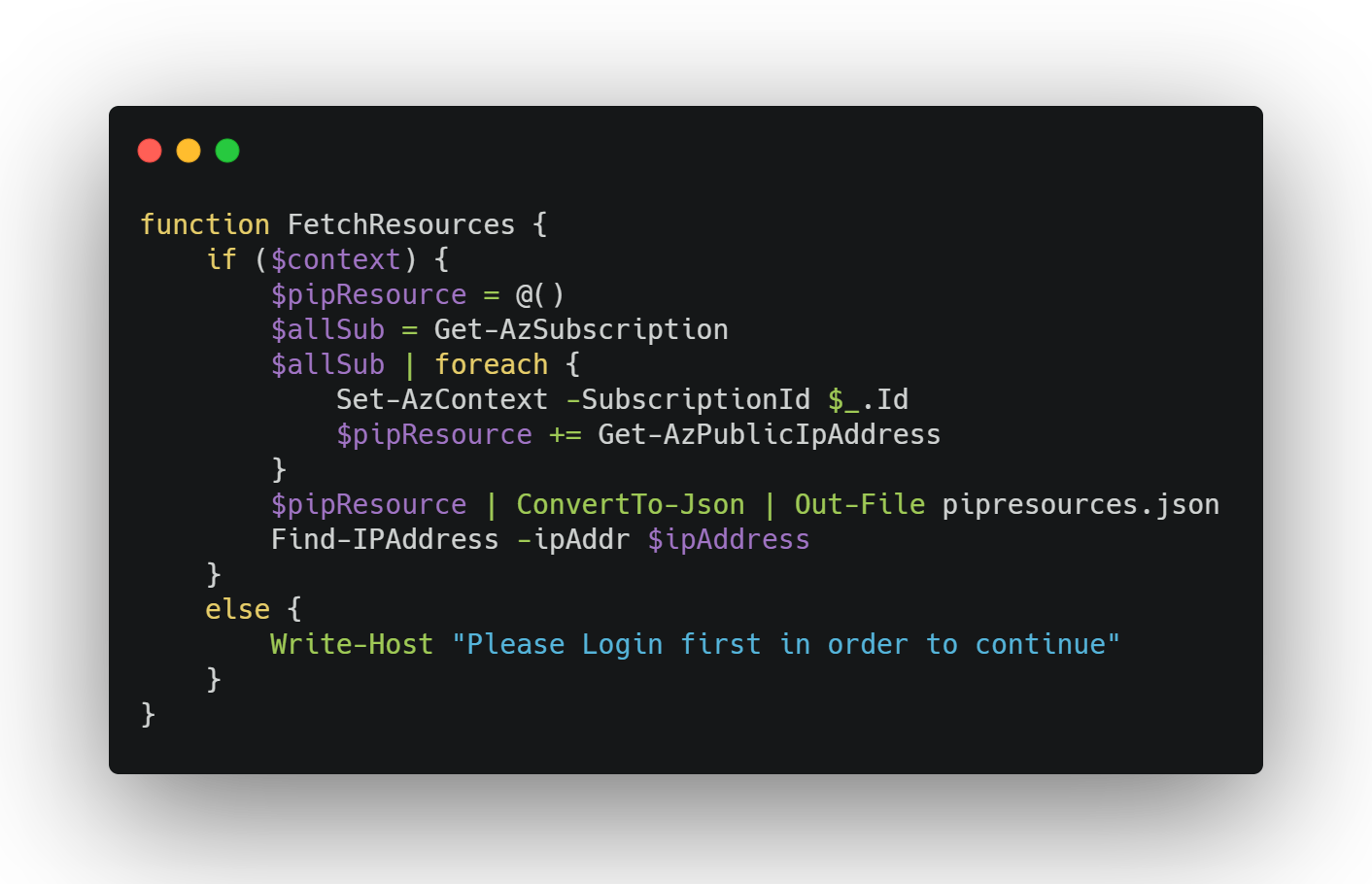
Find-IPAddress
is the core function which does 2 things.
It takes in an IP Address from user that we want to search for check if the cached file already exist from last time under the same folder and then pass the IP to query.
If the file doesn’t exist already, it’ll re-run the FetchResources
and recreate the file with updated information about the resources and perform the query automatically with the IP entered initially.
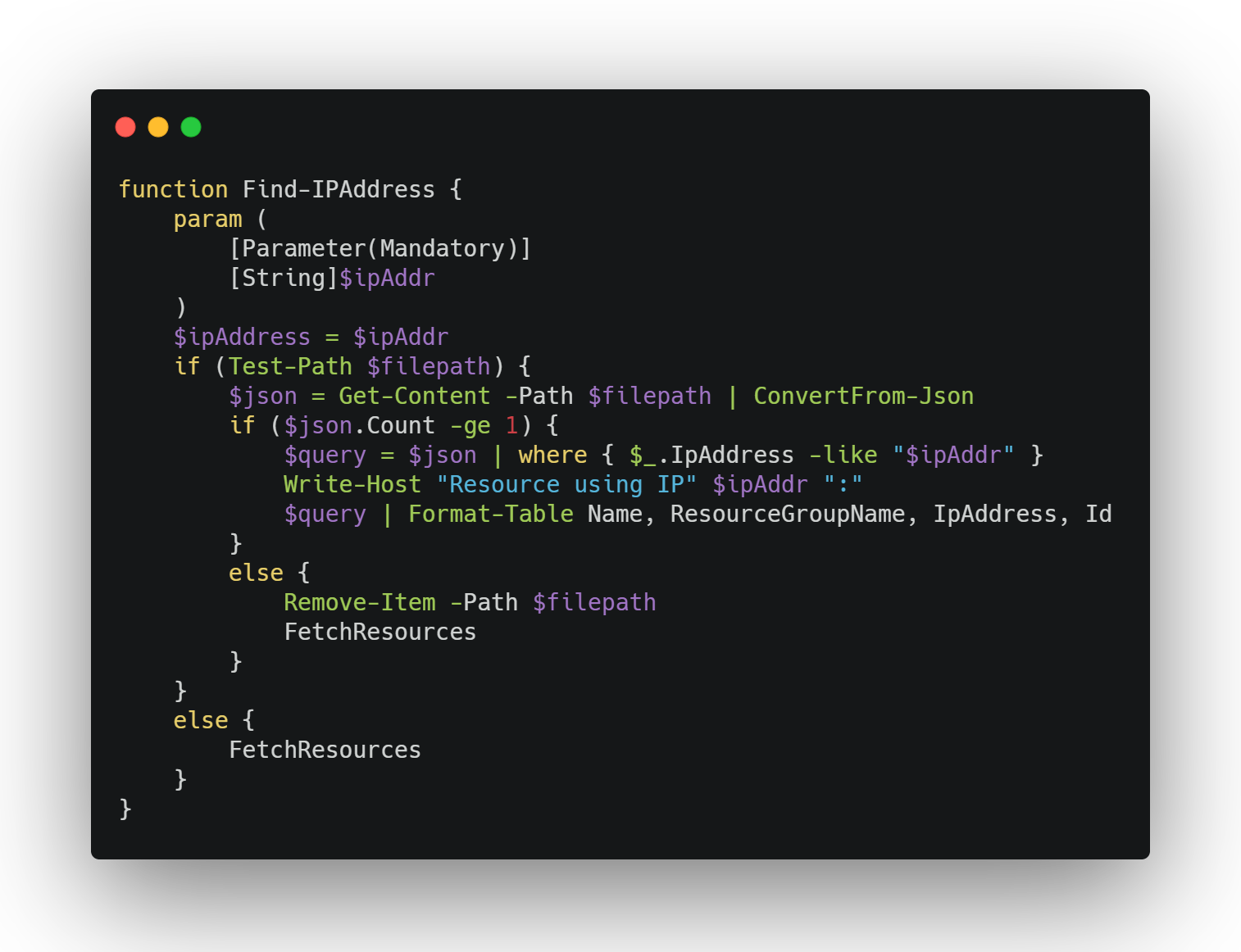
Conclusion
On successfully performing the query and saving the data locally, any following query will be performed against the cached file. If you wish to update the information after you’ve deployed any resources, simple delete the “.json” file or the content within. Script will verify if the data exist or not inside the file and rerun the query based on the observations.
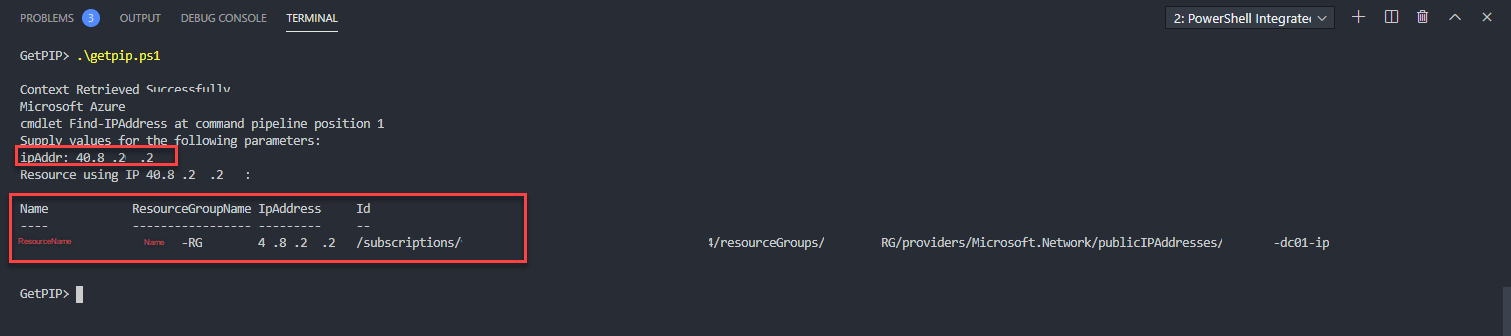
Here’s full script.
$filepath = ".\pipresources.json"
$json = @()
$ipAddress = @()
$context = Get-AzContext
if (!$context) {
Connect-AzAccount
$context = Get-AzContext
}
Write-Host "Context Retrieved Successfully."
Write-Host $context.Name
function FetchResources {
if ($context) {
$pipResource = @()
$allSub = Get-AzSubscription
$allSub | foreach {
Set-AzContext -SubscriptionId $_.Id
$pipResource += Get-AzPublicIpAddress
}
$pipResource | ConvertTo-Json | Out-File pipresources.json
Find-IPAddress -ipAddr $ipAddress
}
else {
Write-Host "Please Login first in order to continue"
}
}
function Find-IPAddress {
param (
[Parameter(Mandatory)]
[String]$ipAddr
)
$ipAddress = $ipAddr
if (Test-Path $filepath) {
$json = Get-Content -Path $filepath | ConvertFrom-Json
if ($json.Count -ge 1) {
$query = $json | where { $_.IpAddress -like "$ipAddr" }
Write-Host "Resource using IP" $ipAddr ":"
$query | Format-Table Name, ResourceGroupName, IpAddress, Id
}
else {
Remove-Item -Path $filepath
FetchResources
}
}
else {
FetchResources
}
}
#run function
Find-IPAddress
You can get the script from GitHub Repo – https://github.com/singhparveen/Get-PIP
Discover more from Parveen Singh
Subscribe to get the latest posts sent to your email.