The system administrator has always been striving for automation. However, for an IT person, repeating the same tasks can become monotonous. Not only does it reduces efficiency, but it can also lead to human errors.
To address the issue of building effective scripts and tools, PowerShell was built using .Net runtime. Due to the flexibility and the powerful command-line execution speed with support for multiple services, PowerShell is loved in the sysadmin and IT world to automate the repeating mundane tasks.
In this article, you’ll discover the basics of PowerShell and what you can do with it.
What is PowerShell?
PowerShell is a fully functional programming and scripting language based on Microsoft .Net framework. It has built-in help and command discovery that lets you discover multiple layers of a framework. So whether you are running Windows, macOS, or Linux, you can use PowerShell for scripting.
As a scripting tool, PowerShell is commonly used for system automation for ongoing maintenance and testing. However, with the extensive functionality, it is getting popular with Azure Cloud to deploy and manage cloud resources programmatically.
What are PowerShell Commands and Cmdlets
PowerShell commands are based on lightweight functions that are designed to perform specific task sets. Therefore, cmdlets are also considered to be building blocks of PowerShell. They are designed to perform one particular action. PowerShell commands are called cmdlets, pronounced as “command-lets.”
Some of the key functionalities of cmdlets are:
- The output of cmdlets is often in the form of an object or array of objects rather than a text string.
- The result of one command can be passed on (pipe) to another command during runtime.
- All the commands are case-insensitive. So, for example,
geT-hElp
andGet-Help
yields the same results.
PowerShell Command Syntax
The PowerShell commands (cmdlets) follow a convention of using a verb and a noun combined with -
(dash) in between them. Therefore, the format looks like this: Verb-Noun
. Thus, while working on any core commands or external library, you will see a consistent pattern on calling the commands.
For example, to query the services running on your computer, you would use Get-Service
. Similarly, you can set
or perform other available actions as well.
Below is a comprehensive list of cmdlet patterns for the verb section of the command:
- Get → get the value of something
- Set → set or update the value of something
- New → create a new entry of something
- Start → start something
- Stop → stop something
- Restart → Restart something
- Out → output something
- Write → write something to terminal or shell
You can always find the list of commands by typing in Get-Command
and review all available commands on your computer:
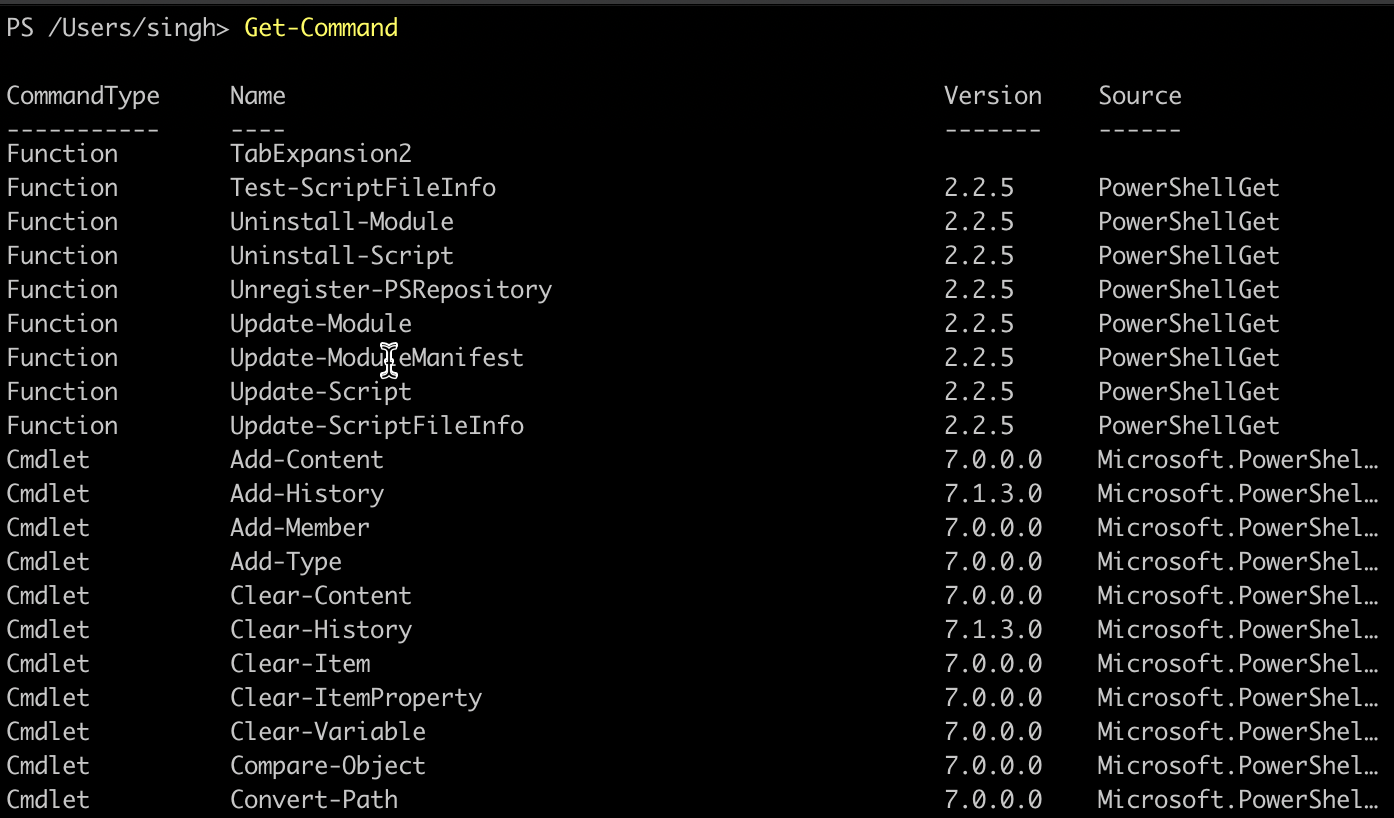
What are PowerShell Modules
PowerShell module is a package that withholds PowerShell members, including functions, cmdlets, provider, workflow, variables, and aliases. The modules are written to solve a single set of problems or target a specific management area on a system.
Running the Get-Module -All
gives you a list of all the installed modules on your computer. Refer to the example where you can see the name of the modules and all the commands each module offers:
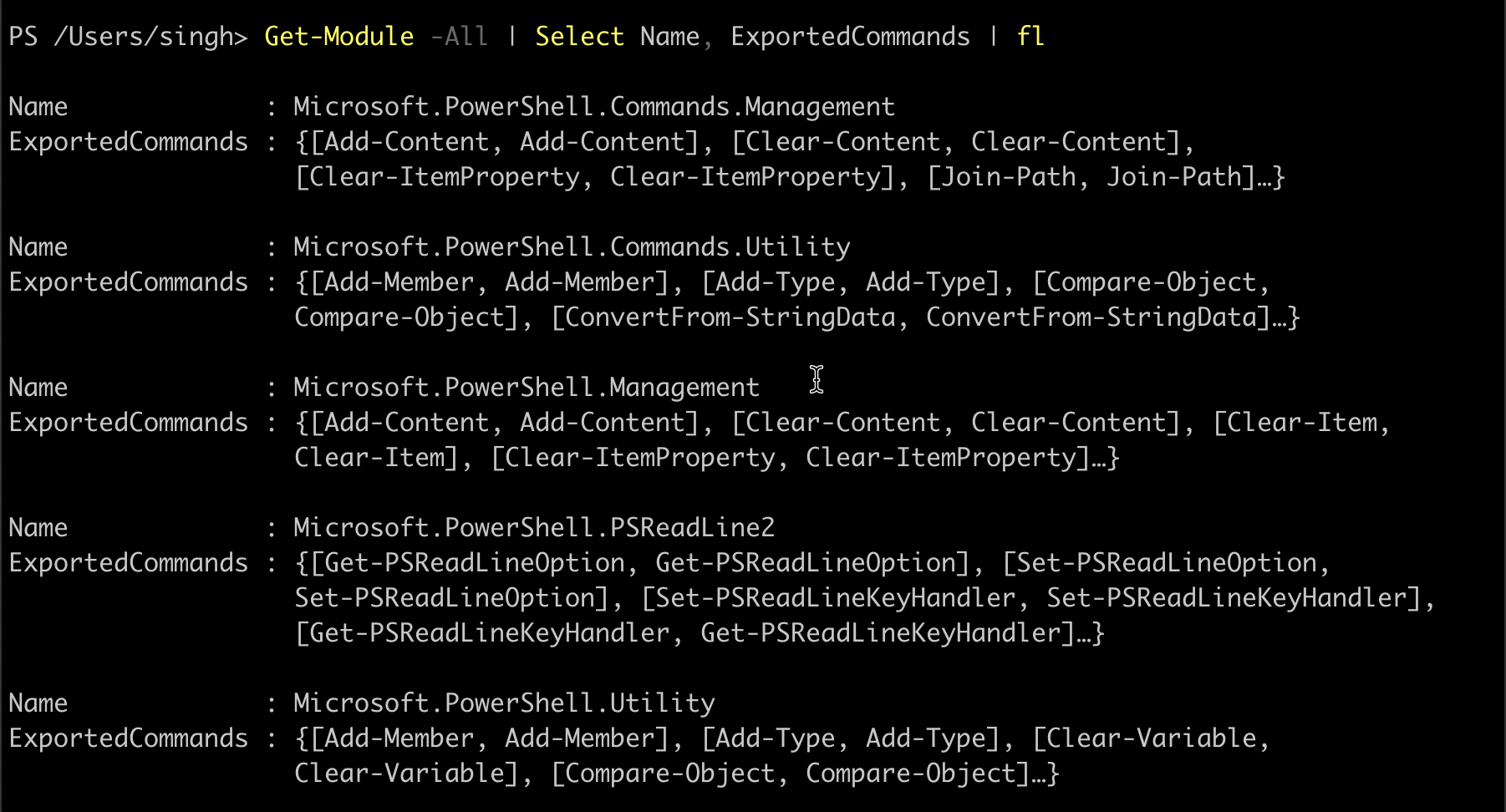
Commands that use PowerShell providers don’t load automatically. Instead, you need to follow a specific process to install and import the modules before they are ready to use. More on this will be covered in a later article.
What is PowerShell Pipeline
PowerShell pipeline is series of commands connected by using a |
(pipe) operator. The output object of one command can serve as an input object for another command. Thus, you can chain multiple commands as long as the data is valid in the following manner:
Command-1 | Command-2 | Command-3
To visualize it with an example, run the following command inside your terminal:
Get-Process | Sort-Object -Property ProcessName -Descending
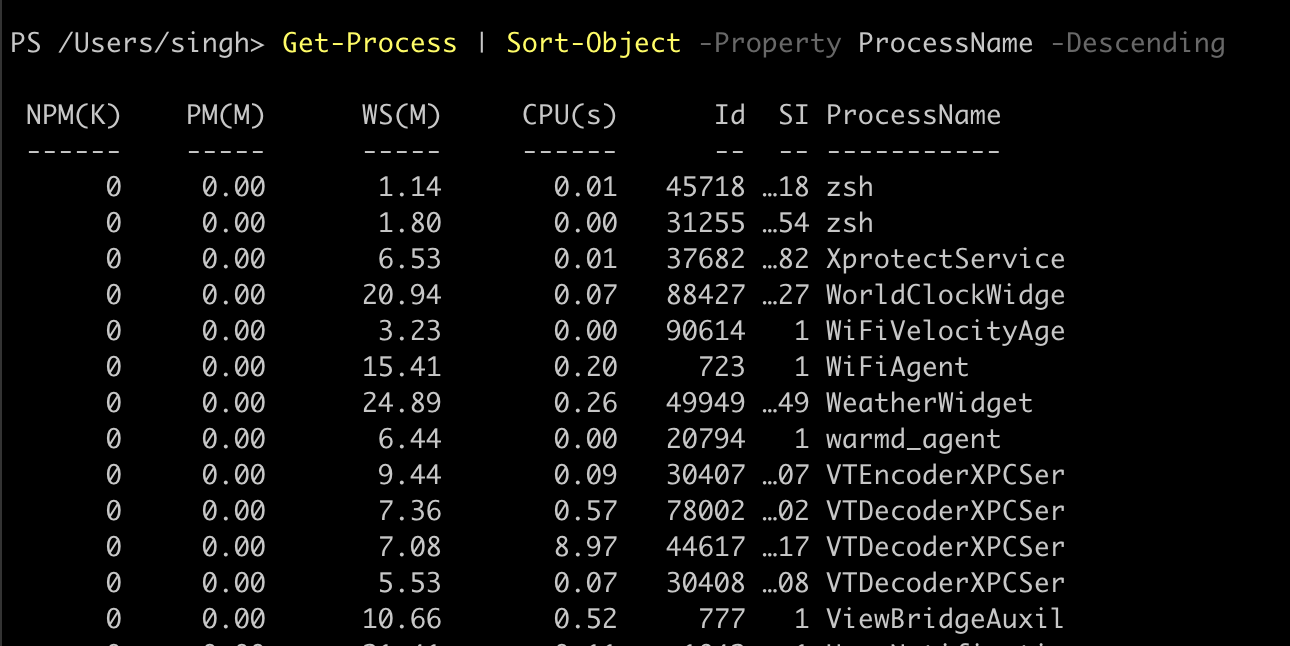
What are PowerShell Objects
With the origin of PowerShell from .Net and being an object-oriented language, every call to PowerShell commands is initializing a class for that module and returning the results. Therefore, the PowerShell objects are instances of classes that have properties and methods you can call.
Here’s an analogy on how to understand more:
- Objects → set of items
- Cmdlets → actions you can perform on those items
- Modules → set of actions you can perform on any item
- Properties → detail about the items
- Methods → instructions for set of items
Common PowerShell Commands
While working with any scripting language, it’s helpful to remember and familiarize yourself with some of the most common and well-known commands. In addition, most of the modules and commands come with specific instructions on how to use the module. Therefore, understanding where to find the information or help resources for any command is key to performing the task.
PowerShell Get-Verb
PowerShell Verb is an interesting concept. The idea of the verb is to define what you are trying to do with the data. Whether it’s read, write, update, or insert, you are expected to follow a certain naming convention.
Run the Get-Verb
command in your terminal to see the list of all the available actions:
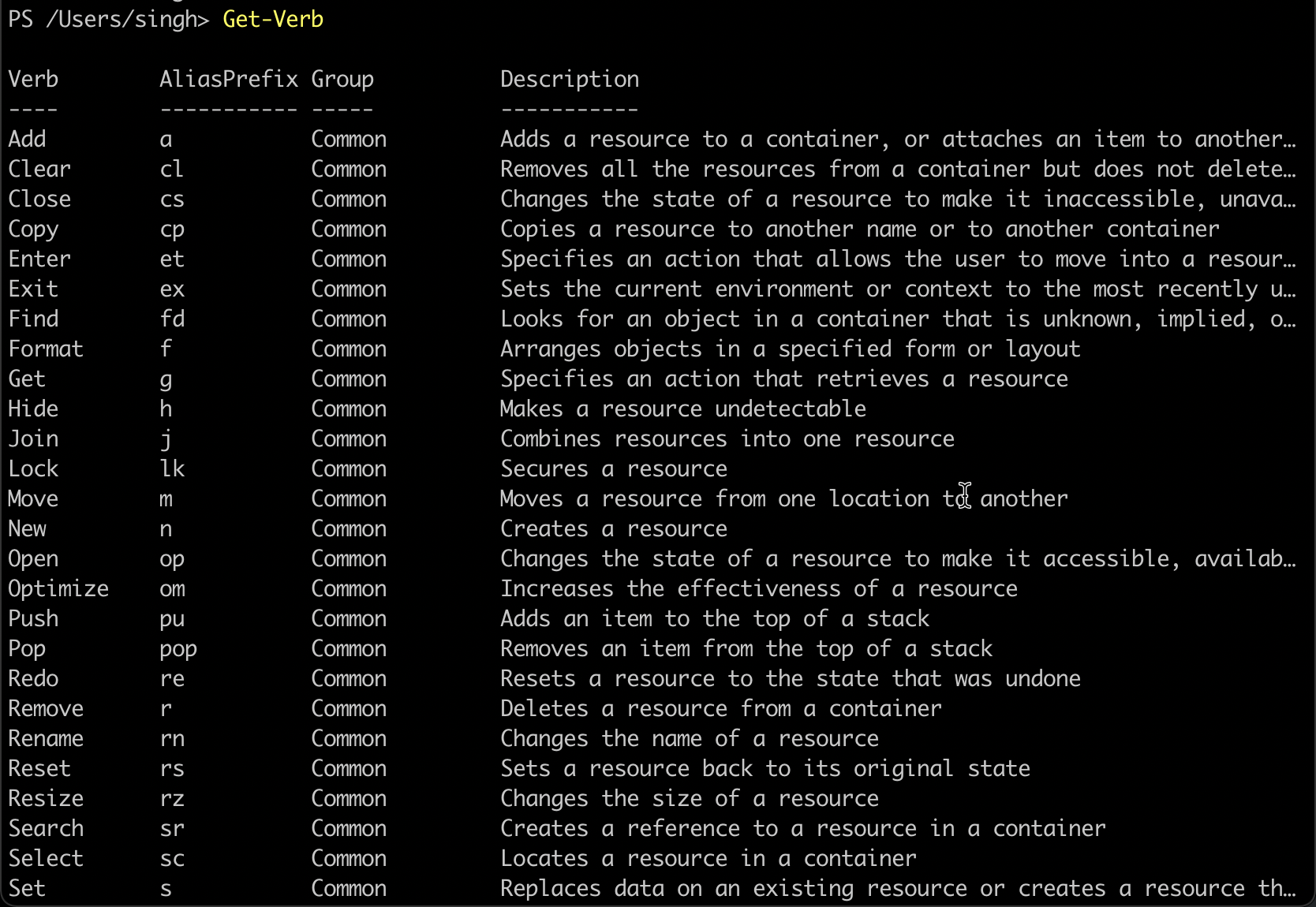
The results may vary in your case. However, notice from the above image, the verbs are grouped (Group) based on their purpose and display an alias (AliasPrefix) that you can use to call the command without typing in the full name.
PowerShell Get-Member
PowerShell Get-Member
command helps you display the list of methods, properties, and objects available for any command. In addition, any command that produces an output of type object can be further called upon with Get-Member to list the information.
Below is a sample command piped with Get-Member
command:
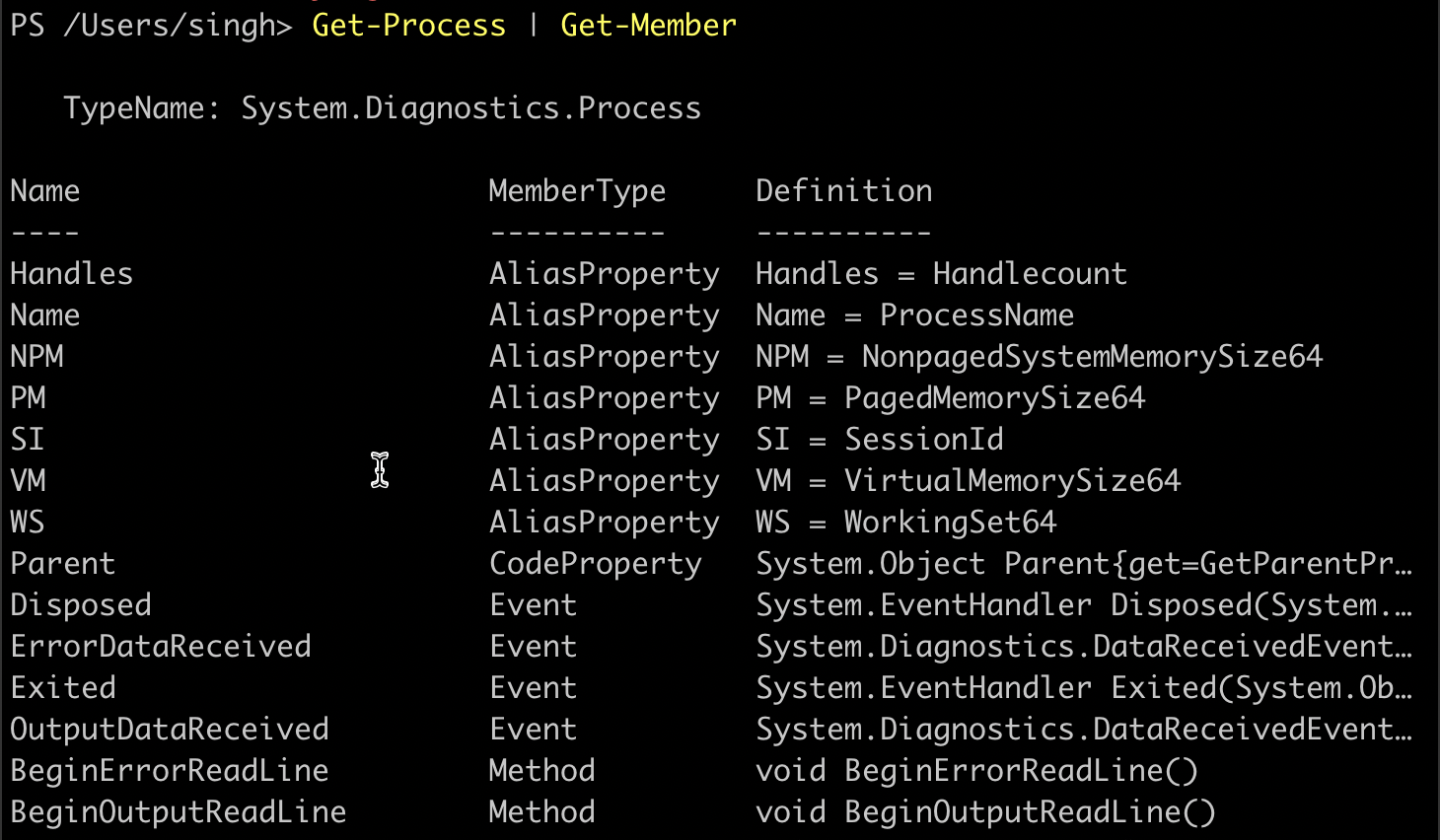
PowerShell Get-Help
Another useful PowerShell command to be aware of in the beginning is the Get-Help
command that can assist you in finding syntax and provide guidance on using all the available commands. For example, the output of Get-Help
looks like this:
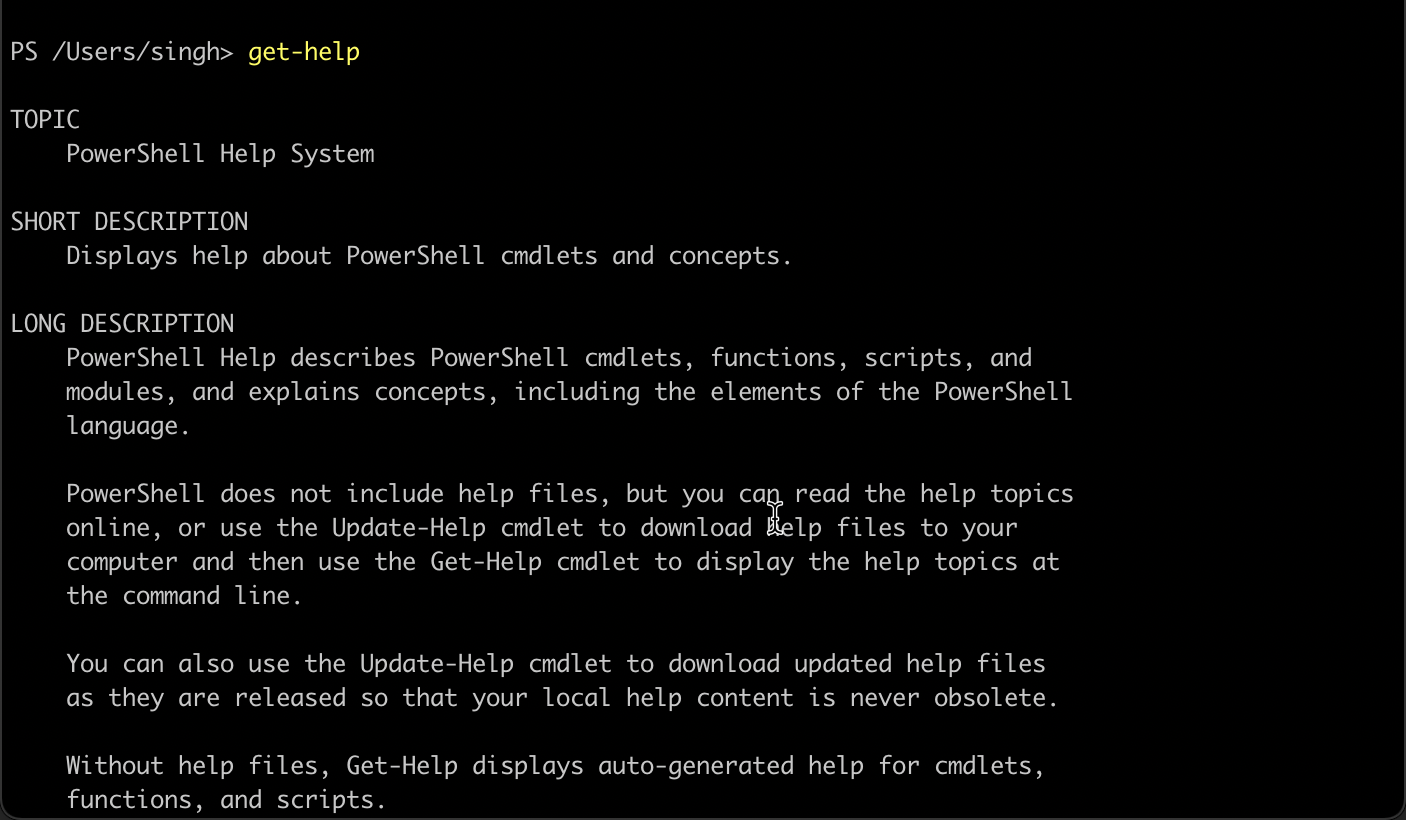
To find the help on any command, type Get-Help
, followed by any command you need help with. In the example above, the call for getting help on Get-Command
looks like this:
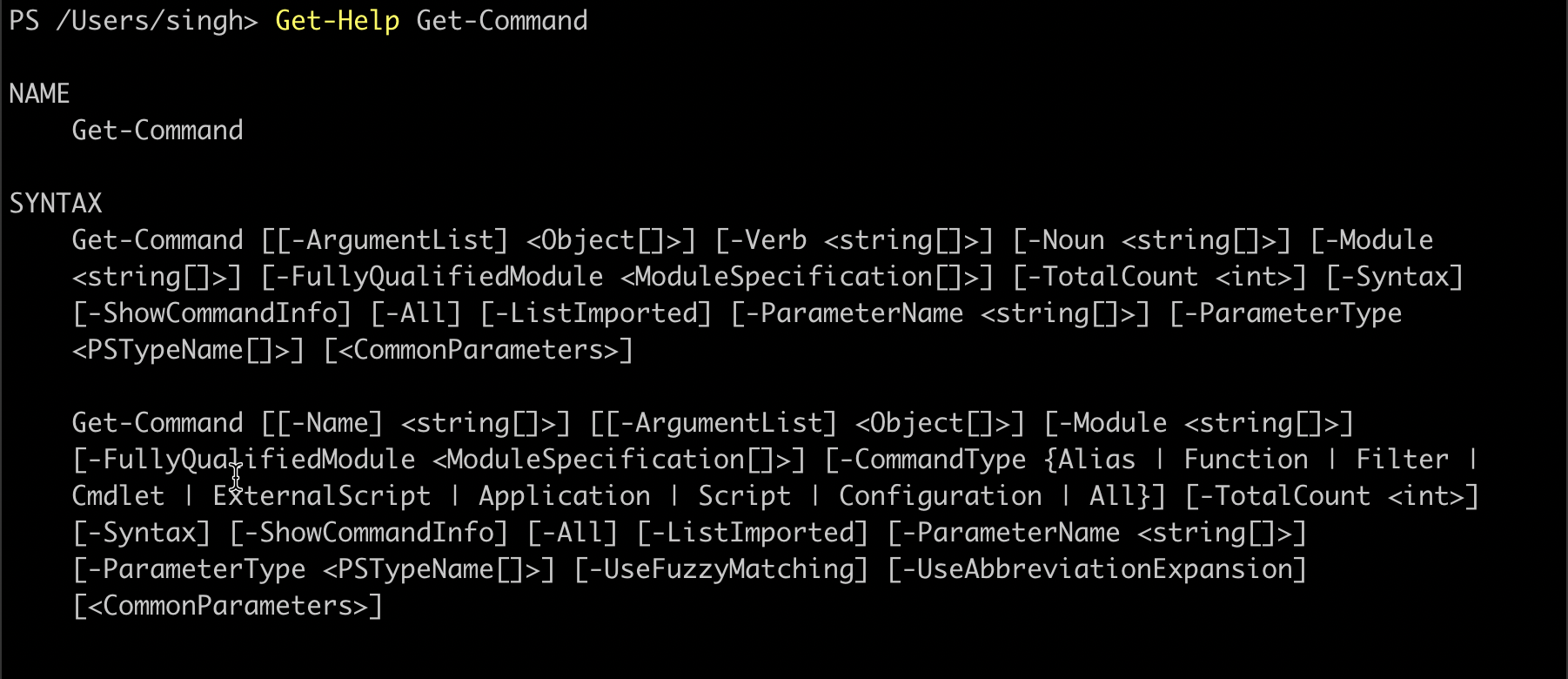
Looking at the output, any command that has more than one parameter set contains a SYNTAX block. The elements in the []
square brackets are optional when running the command.
What are PowerShell Operators
PowerShell operators are commands available out-of-box to perform various operations to analyze and manipulate the data. Some of the common operators include:
- Equality → equal (-eq), not-equal (-ne), greater than (-gt), greater than or equal (-ge), less than (-lt), less than or equal (-le):
PS> 2 -eq 2 # Output: True PS> 2 -eq 3 # Output: False PS> "abc" -eq "abc" # Output: True PS> "abc" -eq "def" # Output: False PS> "abc" -ne "def" # Output: True PS> "abc" -ne "abc" # Output: False
- Matching → -like, -notlike, -match, -notmatch
PS> "PowerShell" -like "*shell" # Output: True PS> "PowerShell" -notlike "*shell" # Output: False PS> "PowerShell" -like "Power?hell" # Output: True PS> "PowerShell" -notlike "Power?hell" # Output: False # Partial match test, showing how differently -match and -like behave PS> "PowerShell" -match 'shell' # Output: True PS> "PowerShell" -like 'shell' # Output: False #Regex syntax test PS> "PowerShell" -match '^Power\\w+' # Output: True PS> 'bag' -notmatch 'b[iou]g' # Output: True
- Containment → -in, -notin, -contains, -notcontains
"abc", "def" -contains "def" # Output: True "abc", "def" -notcontains "def" # Output: False "Windows", "PowerShell" -contains "Shell" # Output: False "Windows", "PowerShell" -notcontains "Shell" # Output: True "abc", "def", "ghi" -contains "abc", "def" # Output: False "abc", "def", "ghi" -notcontains "abc", "def" # Output: True
- Type → -is, -notis
$a = 1 $b = "1" $a -is [int] # Output: True $a -is $b.GetType() # Output: False $b -isnot [int] # Output: True $a -isnot $b.GetType() # Output: True
- Replace → -replace
Conclusion
I hope you find this useful!
Now you know what PowerShell is and understand the basics of how it works. This will go a long way if you plan to use PowerShell for either general system automation or even automating the cloud resources using Azure PowerShell.
In the mean time, check out the other articles in the PowerShell series:
- PowerShell Basics: How to use PowerShell Help?
- PowerShell Basics: Format Command Output as Tables, List, and More
- Azure PowerShell DSC